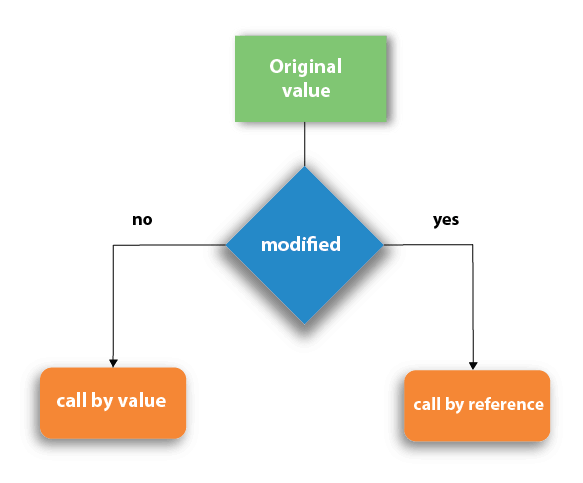
1 - Call by value -
In call by value function, when you make variables and pass them in function, it passes only the value stored in it and it stores that value within other variable during function run. So, your initial value of variables remains same because function uses different variables for it’s work and stores your initial variables value in different variable.
Let inputs be 5 and 7 .#include <iostream> #include <stdlib.h> using namespace std; void swap( int, int ); int main(){ system("cls"); int x, y; cout<<"Enter two numbers\n"; cin>>x>>y; //use spacebar after entering first number cout<<"Numbers before swapping are "<<x<<" and "<<y; swap(x,y); cout<<"\nNumbers after calling swap function are "<<x<<" and "<<y ;} void swap( int a, int b ){ int temp; temp=a; a=b; b=temp; }
In the above example output will be -
You can see that value of numbers before and after swapping remains same.Numbers before swapping are 5 and 7Numbers after swapping are 5 and 7
2 - Call by reference -
In call by reference function, when you make variables and pass them in function, it passes the address stored in it( i.e ).So, variables made within function will store the address of variables passed but not the value(i.e they will act as a pointer). So, when you change the value of variables within function then it will refer to actual variable and change the value of actual variable.
Let inputs be 5 and 7 .#include <iostream> #include <stdlib.h> using namespace std; void swap( int &, int & ); int main(){ system("cls"); int x, y; cout<<"Enter two numbers\n"; cin>>x>>y; //use spacebar after entering first number cout<<"Numbers before swapping are "<<x<<" and "<<y; swap(x,y); cout<<"\nNumbers after calling swap function are "<<x<<" and "<<y ;} void swap( int &a, int &b ){ int temp; temp=a; a=b; b=temp; }
In the above example output will be -
You can see that value of numbers before and after swapping are changed.Numbers before swapping are 5 and 7Numbers after swapping are 7 and 5
Feel free to ask any doubt.
0 Comments